Redux vs Context API Choosing the Best State Management for Your React Project
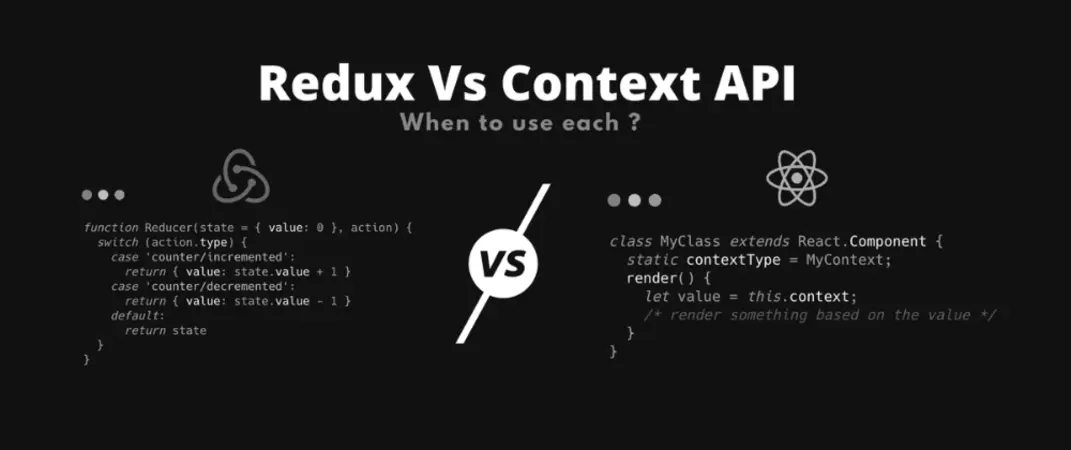
- Published on
- /4 mins read/βββ views
Introduction
Managing state efficiently in a React application is crucial as your project scales. Two popular methods for handling state management are the Context API and Redux. But which one should you choose?
- Context API is built into React and provides a simple way to pass data through components without prop drilling.
- Redux is an external library that offers a centralized store for managing complex application states.
This guide will help you understand the differences between Context API and Redux, their features, and when to use them in your React project.
What is Redux?
Redux is a JavaScript library designed for managing and centralizing application state. It is widely used in large-scale applications to ensure predictable state updates.
Components of Redux
Redux has three core components:
- Store: Holds the application state.
- Actions: Describe state changes.
- Reducers: Define how the state changes based on actions.
Features of Redux
- Single Source of Truth: The entire application state is stored in one location.
- Predictable State Updates: State changes only happen through actions.
- Middleware Support: Enables advanced features like logging and async operations.
Limitations of Redux
- Boilerplate Code: Requires setting up actions, reducers, and store.
- Performance Overhead: Frequent state updates can slow down large apps.
- Steeper Learning Curve: Requires understanding of immutability and functional programming.
How to Set Up Redux in React
Install Redux Toolkit:
npm install @reduxjs/toolkit react-redux
Create a Redux slice:
import { createSlice } from '@reduxjs/toolkit'
const initialState = { count: 0 }
const counterSlice = createSlice({
name: 'counter',
initialState,
reducers: {
increment: (state) => {
state.count += 1
},
decrement: (state) => {
state.count -= 1
},
},
})
export const { increment, decrement } = counterSlice.actions
export default counterSlice.reducer
Set up the store:
import { configureStore } from '@reduxjs/toolkit'
import counterReducer from './counterSlice'
const store = configureStore({
reducer: { counter: counterReducer },
})
export default store
Wrap the app with the Provider
component:
import { Provider } from 'react-redux'
import store from './store'
import App from './App'
export default function Root() {
return (
<Provider store={store}>
<App />
</Provider>
)
}
What is Context API?
The React Context API allows data to be passed through the component tree without manually passing props at every level. It is ideal for small to medium-scale applications.
Components of Context API
- Provider: Supplies data to child components.
- Consumer: Accesses data from the provider.
Advantages of Context API
- Simplifies Prop Drilling: Directly passes data without manual prop forwarding.
- Lightweight and Built-in: No external dependencies needed.
- Easier Setup: Requires less code than Redux.
Limitations of Context API
- Performance Issues: Frequent re-renders can affect performance in large apps.
- No Middleware Support: Lacks built-in tools for handling complex state logic.
How to Use Context API in React
Create a context:
import { createContext } from 'react'
export const AppContext = createContext(null)
Provide the context:
import { useState } from 'react'
import { AppContext } from './AppContext'
import AppComponent from './AppComponent'
function App() {
const [value, setValue] = useState('Hello, Context API')
return (
<AppContext.Provider value={{ value, setValue }}>
<AppComponent />
</AppContext.Provider>
)
}
export default App
Consume the context:
import { useContext } from 'react'
import { AppContext } from './AppContext'
function AppComponent() {
const { value, setValue } = useContext(AppContext)
return (
<div>
<h1>{value}</h1>
<button onClick={() => setValue('Updated Value')}>Update</button>
</div>
)
}
export default AppComponent
Redux vs Context API: A Comparison
Feature | Redux | Context API |
---|---|---|
Approach | Centralized state management | Decentralized state passing |
Performance | Optimized for large apps | May cause re-renders |
Setup Complexity | Requires configuration | Minimal setup |
Middleware Support | Yes | No |
Debugging | Easy with DevTools | Harder in deep trees |
Best Use Case | Large-scale apps | Small to medium apps |
Conclusion
Choosing between Redux and Context API depends on your project size and requirements:
- Use Context API for smaller projects where prop drilling is an issue.
- Use Redux for large-scale applications requiring a centralized state.
Both tools have their strengths, and understanding them will help you make an informed decision in your next React project.
Whatβs your preferred state management approach? Let us know in the comments! π
On this page
Discussion (0)
π Join the conversation!
Log in with GitHub or Google to share your thoughts and connect with the community.