Optimizing MongoDB Indexes for High-Performance Queries
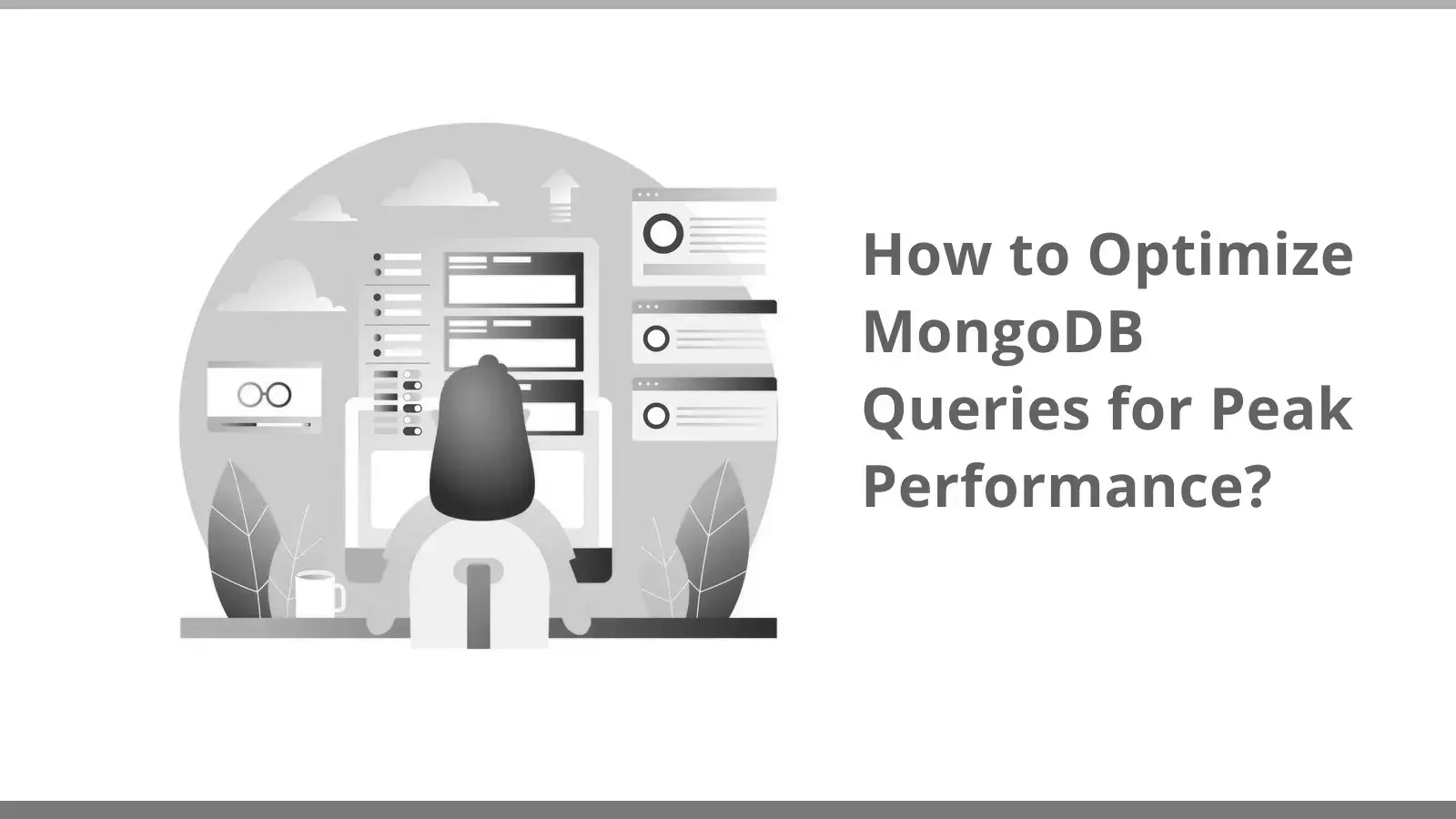
- Published on
- /4 mins read/––– views
Introduction
MongoDB indexes play a crucial role in optimizing query performance and ensuring efficient data retrieval. Properly designed indexes can significantly reduce query execution time and enhance the scalability of your application. This guide will walk you through best practices for using indexes effectively in MongoDB, covering index types, compound indexing strategies, and performance tuning techniques.
What Are Indexes in MongoDB?
Indexes are special data structures that store a small portion of a collection's dataset in an easy-to-traverse form. They help MongoDB locate documents faster compared to a full collection scan.
Check out the official MongoDB documentation for a deep dive into indexes.
Best Practices for MongoDB Index Optimization
1. Always Index Your Collections
Indexing every collection is a fundamental practice for improving query performance. Without indexes, MongoDB scans every document in a collection, leading to slow query execution, especially as data volume grows.
2. Follow the ESR Rule (Equality, Sort, Range) in Compound Indexes
When building compound indexes, use the ESR (Equality, Sort, Range) rule to ensure efficient query execution:
- Equality (
{ field: value }
) - Sort (
sort({ field: 1 })
orsort({ field: -1 })
) - Range Queries (
{ field: { $gt: value } }
,{ field: { $lt: value } }
)
For example, a compound index like { category: 1, date: -1, price: 1 }
optimizes queries that filter by category, sort by date, and use a price range.
3. Use Partial Indexes for Optimized Query Execution
Partial indexes reduce index size by indexing only a subset of documents. This helps in optimizing memory usage and query performance. Use them when queries filter based on specific conditions.
Example:
{ "status": { "$eq": "active" } }
A partial index on status
for active users ensures that only relevant documents are indexed, reducing overhead.
4. Avoid Creating Redundant Indexes
MongoDB automatically supports prefix indexing within compound indexes. If you already have a compound index like:
{ "stock": 1, "date": -1, "price": 1 }
Then its prefixes, such as:
{ stock: 1, date: -1 }
{ stock: 1 }
are implicitly covered. You do not need to create separate indexes for these prefixes.
5. Optimize Index Usage with Projection
Indexing can be further optimized by ensuring queries use projections to fetch only the necessary fields instead of scanning entire documents. This reduces query execution time and memory usage.
Example:
db.products.find({ category: 'electronics' }, { name: 1, price: 1, _id: 0 })
This query will only return the name
and price
fields, improving performance.
6. Avoid Right-Anchored or Unrooted Regular Expressions
Queries using unrooted or right-anchored regex patterns can severely degrade index performance. Avoid these patterns:
/hello/
/.world/
/.*manufacturer$/
Instead, use anchored expressions like:
/^hello/
This ensures that indexes remain effective for search queries.
7. Limit Indexes to a Maximum of 50 Per Collection
While indexes boost read performance, they can negatively impact write performance and memory usage. As a rule of thumb, limit the number of indexes per collection to 50 or fewer to maintain optimal performance.
8. Use Hidden Indexes Before Deleting an Index
Before removing an index, hide it temporarily to measure the performance impact.
db.collection.hideIndex('my_index_1')
Hidden indexes continue to be updated but are not used for queries. If performance drops, you can unhide them instead of recreating them.
$indexStats
9. Monitor Index Usage with To determine which indexes are actively used, run the following command:
db.collection.indexStats()
This helps identify unused indexes that can be safely removed.
10. Leverage Wildcard Indexes for Dynamic Schemas
If your schema is unstructured and fields are dynamically generated at runtime, consider using wildcard indexes.
{ "$**": 1 }
Wildcard indexes allow indexing across multiple unknown fields without predefined keys, making them useful for applications with evolving schemas.
11. Prefer Compound Indexes Over Index Intersections
Instead of relying on multiple single-field indexes, use compound indexes to optimize performance. MongoDB attempts to intersect indexes when multiple indexes are present, but compound indexes generally yield better performance.
Conclusion
Optimizing MongoDB indexes is essential for enhancing query efficiency and maintaining database performance. By following best practices such as the ESR rule, partial indexes, and index monitoring, developers can significantly reduce query execution time while optimizing memory usage.
Further Reading:
Following these strategies ensures that your application remains scalable, efficient, and highly responsive, even as data grows over time.
On this page
- Introduction
- Best Practices for MongoDB Index Optimization
- 1. Always Index Your Collections
- 2. Follow the ESR Rule (Equality, Sort, Range) in Compound Indexes
- 3. Use Partial Indexes for Optimized Query Execution
- 4. Avoid Creating Redundant Indexes
- 5. Optimize Index Usage with Projection
- 6. Avoid Right-Anchored or Unrooted Regular Expressions
- 7. Limit Indexes to a Maximum of 50 Per Collection
- 8. Use Hidden Indexes Before Deleting an Index
- 9. Monitor Index Usage with $indexStats
- 10. Leverage Wildcard Indexes for Dynamic Schemas
- 11. Prefer Compound Indexes Over Index Intersections
- Conclusion
Discussion (0)
🚀 Join the conversation!
Log in with GitHub or Google to share your thoughts and connect with the community.