Step-by-Step Guide: Setting Up a React.js Project with Git and Version Control
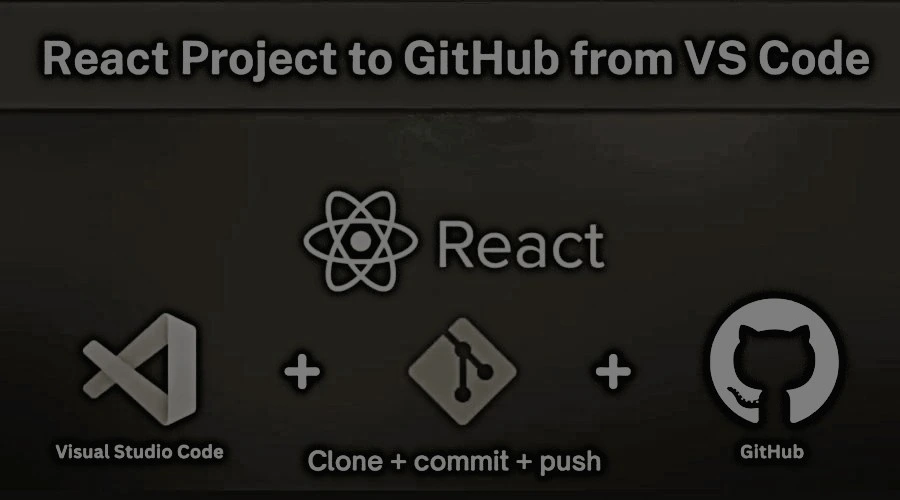
- Published on
- /4 mins read/βββ views
Setting up a React.js project with Git version control is a fundamental skill for modern frontend developers. This guide provides a detailed walkthrough to help you:
- Install Node.js and configure your development environment.
- Create a React.js application using Create React App.
- Set up Git and push your project to GitHub.
- Follow best practices for managing your project repository.
Whether you're a beginner or an experienced developer, this guide ensures you have the tools and knowledge to start your React.js development journey confidently.
π Why Choose React.js for Frontend Development?
React.js is one of the most popular JavaScript libraries for building dynamic, high-performance user interfaces. Hereβs why developers love it:
- Component-Based Architecture: Build reusable and maintainable UI components.
- Fast Rendering: Leverage the Virtual DOM for optimal performance.
- SEO-Friendly: Create single-page applications (SPAs) optimized for search engines, especially with frameworks like Next.js.
- Strong Community Support: React is maintained by Meta (formerly Facebook) and has a vast ecosystem of tools and libraries.
Step 1: Install Node.js and npm
To start with React.js, you need Node.js installed on your system. Node.js provides the runtime environment, while npm (Node Package Manager) helps manage dependencies.
Download Node.js
Choose the appropriate version for your operating system:
Verify Installation
After installation, open your terminal and run:
node -v
npm -v
This will display the installed versions of Node.js and npm.
Step 2: Create a React.js Project
Set up a new React.js application using Create React App, a tool that provides a boilerplate for React projects.
Create Your React App
Run the following commands in your terminal:
npx create-react-app my-react-app
cd my-react-app
This will generate a new React project with essential configurations, including a development server, build tools, and a default project structure.
Start the Development Server
To preview your app, run:
npm start
Your React app will be available at http://localhost:3000/ π
Step 3: Set Up Git and GitHub
Version control is crucial for managing your codebase effectively. Letβs configure Git and push your project to GitHub.
Initialize Git
Inside your project directory, initialize a Git repository:
git init
.gitignore
File
Create a Exclude unnecessary files from your repository by creating a .gitignore
file:
echo "node_modules/\nbuild/\n.env" > .gitignore
Commit Your Code
Add and commit your initial code:
git add .
git commit -m "Initial commit - setup React project"
Create a GitHub Repository
- Log in to GitHub.
- Click New Repository.
- Name your repository
my-react-app
and create it.
Link Local Repository to GitHub
Copy your repository URL and run:
git remote add origin <YOUR_GITHUB_REPOSITORY_URL>
Push Your Code
Push your code to GitHub:
git push -u origin main
Step 4: Git Branching and Best Practices
Using Git branches helps you manage features and bug fixes efficiently.
Create a New Branch
git checkout -b feature-branch
Switch Between Branches
git checkout main
Merge a Branch
git merge feature-branch
Delete a Branch
git branch -d feature-branch
Step 5: Configure Environment Variables in React
To secure sensitive information, use environment variables. Create a .env
file in your project root:
touch .env
Add your variables:
REACT_APP_API_URL=https://api.example.com
Access them in your React app:
const apiUrl = process.env.REACT_APP_API_URL
console.log('API URL:', apiUrl)
π Quick Reference Commands
Hereβs a summary of the commands covered in this guide:
# React project setup
npx create-react-app my-react-app
cd my-react-app
npm start
# Git setup
git init
echo "node_modules/\nbuild/\n.env" > .gitignore
git add .
git commit -m "Initial commit - setup React project"
# Link to GitHub
git remote add origin <YOUR_GITHUB_REPOSITORY_URL>
git push -u origin main
# Git branching
git checkout -b feature-branch
git checkout main
git merge feature-branch
git branch -d feature-branch
π Conclusion
Congratulations! π Youβve successfully set up a React.js project with Git version control. This foundational setup ensures you can develop, manage, and collaborate on your frontend projects efficiently.
π Recommended Resources:
π¬ Have questions or feedback? Drop a comment below! π
On this page
- π Why Choose React.js for Frontend Development?
- Step 1: Install Node.js and npm
- Download Node.js
- Verify Installation
- Step 2: Create a React.js Project
- Create Your React App
- Start the Development Server
- Step 3: Set Up Git and GitHub
- Initialize Git
- Create a .gitignore File
- Commit Your Code
- Create a GitHub Repository
- Link Local Repository to GitHub
- Push Your Code
- Step 4: Git Branching and Best Practices
- Create a New Branch
- Switch Between Branches
- Merge a Branch
- Delete a Branch
- Step 5: Configure Environment Variables in React
- π Quick Reference Commands
- π Conclusion
Discussion (0)
π Join the conversation!
Log in with GitHub or Google to share your thoughts and connect with the community.